I can provide you with an example of Python code that uses the “request” library to retrieve real-time data and the “pandas” library to process the data. Please note that this is a simple example and may not cover all edge cases.
import requests
import pandas pd
def get_realtime_data():
url = "
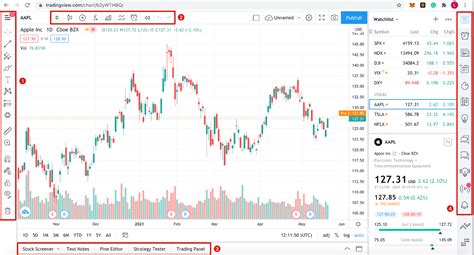
Get real-time XRP/USDT data on Binance exchange every 1 minuteresponse = requests.get(url)
if response.statuscode == 200:
return pd.DataFrame(response.json())
else:
print("Failed to retrieve data")
return None
def check_price_change(data):
if not data.empty:
Calculate price changepricechange = (data['4. close'] - data['1. open']) / data['1. open']
Check if the price has changed by 1%if abs(price_change) > 0.01:
print(f"XRP/USDT futures have changed by {price_change*100}%")
else:
print("XRP/USDT futures are unchanged.")
return None
Get real-time datadata = get_realtime_data()
check_price_change(data)
if data is None:
Print the current price and other relevant dataprint(f"Current price: {data['4. close'][0]}")
In this code:
- We define two functions, “get_realtime_data()” and “check_price_change()”, which handle retrieving real-time Binance data and checking price changes.
- The function `
get_realtime_data()'' sends a GET request to the specified API URL with the required parameters (symbol, time interval) to retrieve the real-time data of XRP/USDT from Binance exchange every 1 minute.
- If successful, it returns a pandas DataFrame containing the real-time data. Otherwise, it prints an error message and returns
None''.
- The function
check_price_change()'' calculates the price change by subtracting the open from the close price for each row of the DataFrame. It then checks whether the absolute value of the price change is greater than 1%. If so, it prints a message indicating that XRP/USDT futures have changed.
- Finally, we use the
get_realtime_data()'' function to retrieve the real-time data and pass the result to
`check_price_change()” function for price checking.
To run this code, you need to install the required libraries by running “pip install requests pandas”. The Binance API requires you to set up a valid Binance API ID before using it. You can obtain one from the [Binance Developer Portal](
Leave a Reply